- JavaScript Introduction
- JavaScript Where To
- JavaScript Output
- JavaScript Statements
- JavaScript Syntax
- JavaScript Comments
- JavaScript Variables
- JavaScript Let
- JavaScript Const
- JavaScript Operators
- JavaScript Assignment
- JavaScript Data Types
- JavaScript Functions
- JavaScript Objects
- JavaScript Events
- JavaScript Strings
- JavaScript String Methods
- JavaScript Numbers
- JavaScript Number Methods
- JavaScript Arrays
- JavaScript Array Const
- JavaScript Array Methods
- JavaScript Sorting Arrays
- JavaScript Array Iteration
- JavaScript Date Objects
- JavaScript Date Formats
- JavaScript Get Date Methods
- JavaScript Set Date Methods
- JavaScript Math Object
- JavaScript Random
- JavaScript Booleans
- JavaScript Comparison And Logical Operators
- JavaScript If Else And Else If
- JavaScript Switch Statement
- JavaScript For Loop
- JavaScript Break And Continue
- JavaScript Type Conversion
- JavaScript Bitwise Operations
- JavaScript Regular Expressions
- JavaScript Errors
- JavaScript Scope
- JavaScript Hoisting
- JavaScript Use Strict
- The JavaScript This Keyword
- JavaScript Arrow Function
- JavaScript Classes
- JavaScript JSON
- JavaScript Debugging
- JavaScript Style Guide
- JavaScript Common Mistakes
- JavaScript Performance
- JavaScript Reserved Words
- JavaScript Versions
- JavaScript History
- JavaScript Forms
- JavaScript Validation API
- JavaScript Objects
- JavaScript Object Properties
- JavaScript Function Definitions
- JavaScript Function Parameters
- JavaScript Function Invocation
- JavaScript Closures
- JavaScript Classes
- Java Script Async
- JavaScript HTML DOM
- The Browser Object Model
- JS Ajax
- JavaScript JSON
- JavaScript Web APIs
- JS Vs JQuery
JavaScript HTML DOM
JavaScript HTML DOM
With the HTML DOM, JavaScript can access and change all the elements of an HTML document.
The HTML DOM (Document Object Model)
When a web page is loaded, the browser creates a Document Object Model of the page.
The HTML DOM model is constructed as a tree of Objects:
The HTML DOM Tree of Objects
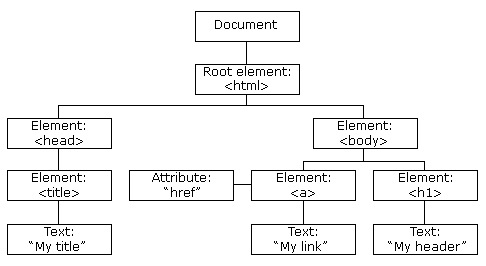
With the object model, JavaScript gets all the power it needs to create dynamic HTML:
- JavaScript can change all the HTML elements in the page
- JavaScript can change all the HTML attributes in the page
- JavaScript can change all the CSS styles in the page
- JavaScript can remove existing HTML elements and attributes
- JavaScript can add new HTML elements and attributes
- JavaScript can react to all existing HTML events in the page
- JavaScript can create new HTML events in the page
What You Will Learn
In the next chapters of this tutorial you will learn:
- How to change the content of HTML elements
- How to change the style (CSS) of HTML elements
- How to react to HTML DOM events
- How to add and delete HTML elements
What is the DOM?
The DOM is a W3C (World Wide Web Consortium) standard.
The DOM defines a standard for accessing documents:
"The W3C Document Object Model (DOM) is a platform and language-neutral interface that allows programs and scripts to dynamically access and update the content, structure, and style of a document."
The W3C DOM standard is separated into 3 different parts:
- Core DOM - standard model for all document types
- XML DOM - standard model for XML documents
- HTML DOM - standard model for HTML documents
What is the HTML DOM?
The HTML DOM is a standard object model and programming interface for HTML. It defines:
- The HTML elements as objects
- The properties of all HTML elements
- The methods to access all HTML elements
- The events for all HTML elements
In other words: The HTML DOM is a standard for how to get, change, add, or delete HTML elements.
Practice Excercise Practice now
JavaScript - HTML DOM Methods
HTML DOM methods are actions you can perform (on HTML Elements).
HTML DOM properties are values (of HTML Elements) that you can set or change.
The DOM Programming Interface
The HTML DOM can be accessed with JavaScript (and with other programming languages).
In the DOM, all HTML elements are defined as objects.
The programming interface is the properties and methods of each object.
A property is a value that you can get or set (like changing the content of an HTML element).
A method is an action you can do (like add or deleting an HTML element).
Example
The following example changes the content (the innerHTML
) of the <p>
element with id="demo"
:
Example
<body>
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML = "Hello World!";
</script>
</body>
</html>
In the example above, getElementById
is a method, while innerHTML
is a property.
The getElementById Method
The most common way to access an HTML element is to use the id
of the element.
In the example above the getElementById
method used id="demo"
to find the element.
The innerHTML Property
The easiest way to get the content of an element is by using the innerHTML
property.
The innerHTML
property is useful for getting or replacing the content of HTML elements.
The innerHTML
property can be used to get or change any HTML element, including <html>
and <body>
.
Practice Excercise Practice now
JavaScript HTML DOM Document
The HTML DOM document object is the owner of all other objects in your web page.
The HTML DOM Document Object
The document object represents your web page.
If you want to access any element in an HTML page, you always start with accessing the document object.
Below are some examples of how you can use the document object to access and manipulate HTML.
Finding HTML Elements
Method | Description |
---|---|
document.getElementById(id) | Find an element by element id |
document.getElementsByTagName(name) | Find elements by tag name |
document.getElementsByClassName(name) | Find elements by class name |
Changing HTML Elements
Property | Description |
---|---|
element.innerHTML = new html content | Change the inner HTML of an element |
element.attribute = new value | Change the attribute value of an HTML element |
element.style.property = new style | Change the style of an HTML element |
Method | Description |
element.setAttribute(attribute, value) | Change the attribute value of an HTML element |
Adding and Deleting Elements
Method | Description |
---|---|
document.createElement(element) | Create an HTML element |
document.removeChild(element) | Remove an HTML element |
document.appendChild(element) | Add an HTML element |
document.replaceChild(new, old) | Replace an HTML element |
document.write(text) | Write into the HTML output stream |
Adding Events Handlers
Method | Description |
---|---|
document.getElementById(id).onclick = function(){code} | Adding event handler code to an onclick event |
Finding HTML Objects
The first HTML DOM Level 1 (1998), defined 11 HTML objects, object collections, and properties. These are still valid in HTML5.
Later, in HTML DOM Level 3, more objects, collections, and properties were added.
Property | Description | DOM |
---|---|---|
document.anchors | Returns all <a> elements that have a name attribute | 1 |
document.applets | Deprecated | 1 |
document.baseURI | Returns the absolute base URI of the document | 3 |
document.body | Returns the <body> element | 1 |
document.cookie | Returns the document's cookie | 1 |
document.doctype | Returns the document's doctype | 3 |
document.documentElement | Returns the <html> element | 3 |
document.documentMode | Returns the mode used by the browser | 3 |
document.documentURI | Returns the URI of the document | 3 |
document.domain | Returns the domain name of the document server | 1 |
document.domConfig | Obsolete. | 3 |
document.embeds | Returns all <embed> elements | 3 |
document.forms | Returns all <form> elements | 1 |
document.head | Returns the <head> element | 3 |
document.images | Returns all <img> elements | 1 |
document.implementation | Returns the DOM implementation | 3 |
document.inputEncoding | Returns the document's encoding (character set) | 3 |
document.lastModified | Returns the date and time the document was updated | 3 |
document.links | Returns all <area> and <a> elements that have a href attribute | 1 |
document.readyState | Returns the (loading) status of the document | 3 |
document.referrer | Returns the URI of the referrer (the linking document) | 1 |
document.scripts | Returns all <script> elements | 3 |
document.strictErrorChecking | Returns if error checking is enforced | 3 |
document.title | Returns the <title> element | 1 |
document.URL | Returns the complete URL of the document | 1 |
Practice Excercise Practice now
JavaScript HTML DOM Elements
This page teaches you how to find and access HTML elements in an HTML page.
Finding HTML Elements
Often, with JavaScript, you want to manipulate HTML elements.
To do so, you have to find the elements first. There are several ways to do this:
- Finding HTML elements by id
- Finding HTML elements by tag name
- Finding HTML elements by class name
- Finding HTML elements by CSS selectors
- Finding HTML elements by HTML object collections
Finding HTML Element by Id
The easiest way to find an HTML element in the DOM, is by using the element id.
This example finds the element with id="intro"
:
Example
If the element is found, the method will return the element as an object (in myElement).
If the element is not found, myElement will contain null
.
Finding HTML Elements by Tag Name
This example finds all <p>
elements:
Example
This example finds the element with id="main"
, and then finds all <p>
elements inside "main"
:
Example
const y = x.getElementsByTagName("p");
Finding HTML Elements by Class Name
If you want to find all HTML elements with the same class name, use getElementsByClassName()
.
This example returns a list of all elements with class="intro"
.
Example
Finding elements by class name does not work in Internet Explorer 8 and earlier versions.
Finding HTML Elements by CSS Selectors
If you want to find all HTML elements that match a specified CSS selector (id, class names, types, attributes, values of attributes, etc), use the querySelectorAll()
method.
This example returns a list of all <p>
elements with class="intro"
.
Example
The querySelectorAll()
method does not work in Internet Explorer 8 and earlier versions.
Finding HTML Elements by HTML Object Collections
This example finds the form element with id="frm1"
, in the forms collection, and displays all element values:
Example
let text = "";
for (let i = 0; i < x.length; i++) {
text += x.elements[i].value + "<br>";
}
document.getElementById("demo").innerHTML = text;
The following HTML objects (and object collections) are also accessible:
- document.anchors
- document.body
- document.documentElement
- document.embeds
- document.forms
- document.head
- document.images
- document.links
- document.scripts
- document.title
Practice Excercise Practice now
JavaScript HTML DOM - Changing HTML
The HTML DOM allows JavaScript to change the content of HTML elements.
Changing HTML Content
The easiest way to modify the content of an HTML element is by using the innerHTML
property.
To change the content of an HTML element, use this syntax:
document.getElementById(id).innerHTML = new HTMLThis example changes the content of a <p>
element:
Example
<body>
<p id="p1">Hello World!</p>
<script>
document.getElementById("p1").innerHTML = "New text!";
</script>
</body>
</html>
Example explained:
- The HTML document above contains a
<p>
element withid="p1"
- We use the HTML DOM to get the element with
id="p1"
- A JavaScript changes the content (
innerHTML
) of that element to "New text!"
This example changes the content of an <h1>
element:
Example
<html>
<body>
<h1 id="id01">Old Heading</h1>
<script>
const element = document.getElementById("id01");
element.innerHTML = "New Heading";
</script>
</body>
</html>
Example explained:
- The HTML document above contains an
<h1>
element withid="id01"
- We use the HTML DOM to get the element with
id="id01"
- A JavaScript changes the content (
innerHTML
) of that element to "New Heading"
Changing the Value of an Attribute
To change the value of an HTML attribute, use this syntax:
document.getElementById(id).attribute = new valueThis example changes the value of the src attribute of an <img>
element:
Example
<html>
<body>
<img id="myImage" src="smiley.gif">
<script>
document.getElementById("myImage").src = "landscape.jpg";
</script>
</body>
</html>
Example explained:
- The HTML document above contains an
<img>
element withid="myImage"
- We use the HTML DOM to get the element with
id="myImage"
- A JavaScript changes the
src
attribute of that element from "smiley.gif" to "landscape.jpg"
Dynamic HTML content
JavaScript can create dynamic HTML content:
Date : Mon Jun 14 2021 13:10:07 GMT+0530 (India Standard Time)
Example
<html>
<body>
<script>
document.getElementById("demo").innerHTML = "Date : " + Date(); </script>
</body>
</html>
document.write()
In JavaScript, document.write()
can be used to write directly to the HTML output stream:
Example
<html>
<body>
<p>Bla bla bla</p>
<script>
document.write(Date());
</script>
<p>Bla bla bla</p>
</body>
</html>
Never use document.write()
after the document is loaded. It will overwrite the document.
Practice Excercise Practice now
JavaScript HTML DOM - Changing CSS
The HTML DOM allows JavaScript to change the style of HTML elements.
Changing HTML Style
To change the style of an HTML element, use this syntax:
document.getElementById(id).style.property = new styleThe following example changes the style of a <p>
element:
Example
<body>
<p id="p2">Hello World!</p>
<script>
document.getElementById("p2").style.color = "blue";
</script>
</body>
</html>
Using Events
The HTML DOM allows you to execute code when an event occurs.
Events are generated by the browser when "things happen" to HTML elements:
- An element is clicked on
- The page has loaded
- Input fields are changed
You will learn more about events in the next chapter of this tutorial.
This example changes the style of the HTML element with id="id1"
, when the user clicks a button:
Example
<html>
<body>
<h1 id="id1">My Heading 1</h1>
<button type="button"
onclick="document.getElementById('id1').style.color = 'red'">
Click Me!</button>
</body>
</html>
Practice Excercise Practice now
JavaScript HTML DOM Animation
Learn to create HTML animations using JavaScript.
A Basic Web Page
To demonstrate how to create HTML animations with JavaScript, we will use a simple web page:
Example
<html>
<body>
<h1>My First JavaScript Animation</h1>
<div id="animation">My animation will go here</div>
</body>
</html>
Create an Animation Container
All animations should be relative to a container element.
Example
<div id ="animate">My animation will go here</div>
</div>
Style the Elements
The container element should be created with style = "position: relative
".
The animation element should be created with style = "position: absolute
".
Example
width: 400px;
height: 400px;
position: relative;
background: yellow;
}
#animate {
width: 50px;
height: 50px;
position: absolute;
background: red;
}
Animation Code
JavaScript animations are done by programming gradual changes in an element's style.
The changes are called by a timer. When the timer interval is small, the animation looks continuous.
The basic code is:
Example
function frame() {
if (/* test for finished */) {
clearInterval(id);
} else {
/* code to change the element style */
}
}
Create the Full Animation Using JavaScript
Example
let id = null;
const elem = document.getElementById("animate");
let pos = 0;
clearInterval(id);
id = setInterval(frame, 5);
function frame() {
if (pos == 350) {
clearInterval(id);
} else {
pos++;
elem.style.top = pos + 'px';
elem.style.left = pos + 'px';
}
}
}
Practice Excercise Practice now
JavaScript HTML DOM Events
Reacting to Events
A JavaScript can be executed when an event occurs, like when a user clicks on an HTML element.
To execute code when a user clicks on an element, add JavaScript code to an HTML event attribute:
onclick=JavaScriptExamples of HTML events:
- When a user clicks the mouse
- When a web page has loaded
- When an image has been loaded
- When the mouse moves over an element
- When an input field is changed
- When an HTML form is submitted
- When a user strokes a key
In this example, the content of the <h1>
element is changed when a user clicks on it:
Example
<html>
<body>
<h1 onclick="this.innerHTML = 'Ooops!'">Click on this text!</h1>
</body>
</html>
In this example, a function is called from the event handler:
Example
<html>
<body>
<h1 onclick="changeText(this)">Click on this text!</h1>
<script>
function changeText(id) {
id.innerHTML = "Ooops!";
}
</script>
</body>
</html>
HTML Event Attributes
To assign events to HTML elements you can use event attributes.
Example
Assign an onclick event to a button element:
In the example above, a function named
displayDate
will be executed when the button is clicked.
Assign Events Using the HTML DOM
The HTML DOM allows you to assign events to HTML elements using JavaScript:
Example
Assign an onclick event to a button element:
document.getElementById("myBtn").onclick = displayDate;
</script>
displayDate
is assigned to an HTML element with the id="myBtn"
.
The function will be executed when the button is clicked.
The onload and onunload Events
The onload
and onunload
events are triggered when the user enters or leaves the page.
The onload
event can be used to check the visitor's browser type and browser version, and load the proper version of the web page based on the information.
The onload
and onunload
events can be used to deal with cookies.
Example
The onchange Event
The onchange
event is often used in combination with validation of input fields.
Below is an example of how to use the onchange. The upperCase()
function will be called when a user changes the content of an input field.
Example
The onmouseover and onmouseout Events
The onmouseover
and onmouseout
events can be used to trigger a function when the user mouses over, or out of, an HTML element:
The onmousedown, onmouseup and onclick Events
The onmousedown
, onmouseup
, and onclick
events are all parts of a mouse-click. First when a mouse-button is clicked, the onmousedown event is triggered, then, when the mouse-button is released, the onmouseup event is triggered, finally, when the mouse-click is completed, the onclick event is triggered.
More Examples
onmousedown and onmouseup
Change an image when a user holds down the mouse button.
onload
Display an alert box when the page has finished loading.
onfocus
Change the background-color of an input field when it gets focus.
Mouse Events
Change the color of an element when the cursor moves over it.
Practice Excercise Practice now
JavaScript HTML DOM EventListener
The addEventListener() method
Example
Add an event listener that fires when a user clicks a button:
The addEventListener()
method attaches an event handler to the specified element.
The addEventListener()
method attaches an event handler to an element without overwriting existing event handlers.
You can add many event handlers to one element.
You can add many event handlers of the same type to one element, i.e two "click" events.
You can add event listeners to any DOM object not only HTML elements. i.e the window object.
The addEventListener()
method makes it easier to control how the event reacts to bubbling.
When using the addEventListener()
method, the JavaScript is separated from the HTML markup, for better readability and allows you to add event listeners even when you do not control the HTML markup.
You can easily remove an event listener by using the removeEventListener()
method.
Syntax
The first parameter is the type of the event (like "click
" or "mousedown
" or any other HTML DOM Event.)
The second parameter is the function we want to call when the event occurs.
The third parameter is a boolean value specifying whether to use event bubbling or event capturing. This parameter is optional.
Note that you don't use the "on" prefix for the event; use "click
" instead of "onclick
".
Add an Event Handler to an Element
Example
Alert "Hello World!" when the user clicks on an element:
You can also refer to an external "named" function:
Example
Alert "Hello World!" when the user clicks on an element:
function myFunction() {
alert ("Hello World!");
}
Add Many Event Handlers to the Same Element
The addEventListener()
method allows you to add many events to the same element, without overwriting existing events:
Example
element.addEventListener("click", mySecondFunction);
You can add events of different types to the same element:
Example
element.addEventListener("click", mySecondFunction);
element.addEventListener("mouseout", myThirdFunction);
Add an Event Handler to the window Object
The addEventListener()
method allows you to add event listeners on any HTML DOM object such as HTML elements, the HTML document, the window object, or other objects that support events, like the xmlHttpRequest
object.
Example
Add an event listener that fires when a user resizes the window:
document.getElementById("demo").innerHTML = sometext;
});
Passing Parameters
When passing parameter values, use an "anonymous function" that calls the specified function with the parameters:
Example
Event Bubbling or Event Capturing?
There are two ways of event propagation in the HTML DOM, bubbling and capturing.
In bubbling the inner most element's event is handled first and then the outer: the <p>
element's click event is handled first, then the <div>
element's click event.
In capturing the outer most element's event is handled first and then the inner: the <div>
element's click event will be handled first, then the <p>
element's click event.
With the addEventListener()
method you can specify the propagation type by using the "useCapture" parameter:
The default value is false, which will use the bubbling propagation, when the value is set to true, the event uses the capturing propagation.
Example
document.getElementById("myDiv").addEventListener("click", myFunction, true);
The removeEventListener() method
The removeEventListener()
method removes event handlers that have been attached with the addEventListener() method:
Example
Practice Excercise Practice now
JavaScript HTML DOM Navigation
With the HTML DOM, you can navigate the node tree using node relationships.
DOM Nodes
According to the W3C HTML DOM standard, everything in an HTML document is a node:
- The entire document is a document node
- Every HTML element is an element node
- The text inside HTML elements are text nodes
- Every HTML attribute is an attribute node (deprecated)
- All comments are comment nodes
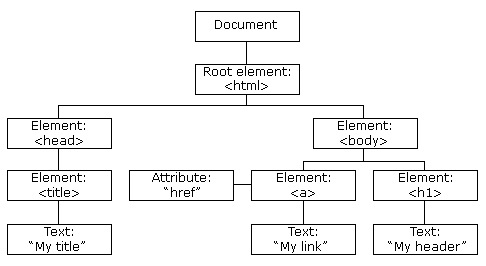
With the HTML DOM, all nodes in the node tree can be accessed by JavaScript.
New nodes can be created, and all nodes can be modified or deleted.
Node Relationships
The nodes in the node tree have a hierarchical relationship to each other.
The terms parent, child, and sibling are used to describe the relationships.
- In a node tree, the top node is called the root (or root node)
- Every node has exactly one parent, except the root (which has no parent)
- A node can have a number of children
- Siblings (brothers or sisters) are nodes with the same parent
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
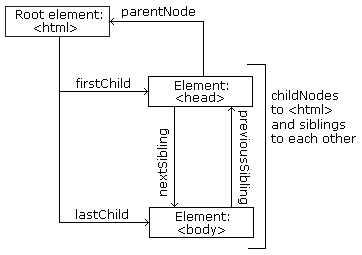
From the HTML above you can read:
<html>
is the root node<html>
has no parents<html>
is the parent of<head>
and<body>
<head>
is the first child of<html>
<body>
is the last child of<html>
and:
<head>
has one child:<title>
<title>
has one child (a text node): "DOM Tutorial"<body>
has two children:<h1>
and<p>
<h1>
has one child: "DOM Lesson one"<p>
has one child: "Hello world!"<h1>
and<p>
are siblings
Navigating Between Nodes
You can use the following node properties to navigate between nodes with JavaScript:
parentNode
childNodes[nodenumber]
firstChild
lastChild
nextSibling
previousSibling
Child Nodes and Node Values
A common error in DOM processing is to expect an element node to contain text.
Example:
<title>
(in the example above) does not contain text.innerHTML
property:nodeValue
of the first child:<h1>
element and copies it into a <p>
element:Example
<body>
<h1 id="id01">My First Page</h1>
<p id="id02"></p>
<script>
document.getElementById("id02").innerHTML = document.getElementById("id01").innerHTML;
</script>
</body>
</html>
Example
<body>
<h1 id="id01">My First Page</h1>
<p id="id02"></p>
<script>
document.getElementById("id02").innerHTML = document.getElementById("id01").firstChild.nodeValue;
</script>
</body>
</html>
Example
<body>
<h1 id="id01">My First Page</h1>
<p id="id02">Hello!</p>
<script>
document.getElementById("id02").innerHTML = document.getElementById("id01").childNodes[0].nodeValue;
</script>
</body>
</html>
InnerHTML
In this tutorial we use the innerHTML property to retrieve the content of an HTML element.
However, learning the other methods above is useful for understanding the tree structure and the navigation of the DOM.
DOM Root Nodes
There are two special properties that allow access to the full document:
document.body
- The body of the documentdocument.documentElement
- The full document
Example
<body>
<h2>JavaScript HTMLDOM</h2>
<p>Displaying document.body</p>
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML = document.body.innerHTML;
</script>
</body>
</html>
Example
<body>
<h2>JavaScript HTMLDOM</h2>
<p>Displaying document.documentElement</p>
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML = document.documentElement.innerHTML;
</script>
</body>
</html>
The nodeName Property
The nodeName
property specifies the name of a node.
- nodeName is read-only
- nodeName of an element node is the same as the tag name
- nodeName of an attribute node is the attribute name
- nodeName of a text node is always #text
- nodeName of the document node is always #document
Example
<p id="id02"></p>
<script>
document.getElementById("id02").innerHTML = document.getElementById("id01").nodeName;
</script>
Note: nodeName
always contains the uppercase tag name of an HTML element.
The nodeValue Property
The nodeValue
property specifies the value of a node.
- nodeValue for element nodes is
null
- nodeValue for text nodes is the text itself
- nodeValue for attribute nodes is the attribute value
The nodeType Property
The nodeType
property is read only. It returns the type of a node.
Example
<p id="id02"></p>
<script>
document.getElementById("id02").innerHTML = document.getElementById("id01").nodeType;
</script>
The most important nodeType properties are:
Node | Type | Example |
---|---|---|
ELEMENT_NODE | 1 | <h1 class="heading">mytat</h1> |
ATTRIBUTE_NODE | 2 | class = "heading" (deprecated) |
TEXT_NODE | 3 | mytat |
COMMENT_NODE | 8 | <!-- This is a comment --> |
DOCUMENT_NODE | 9 | The HTML document itself (the parent of <html>) |
DOCUMENT_TYPE_NODE | 10 | <!Doctype html> |
Type 2 is deprecated in the HTML DOM (but works). It is not deprecated in the XML DOM.
Practice Excercise Practice now
JavaScript HTML DOM Elements (Nodes)
Adding and Removing Nodes (HTML Elements)
Creating New HTML Elements (Nodes)
To add a new element to the HTML DOM, you must create the element (element node) first, and then append it to an existing element.
Example
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
<script>
const para = document.createElement("p");
const node = document.createTextNode("This is new.");
para.appendChild(node);
const element = document.getElementById("div1");
element.appendChild(para);
</script>
Example Explained
This code creates a new <p>
element:
To add text to the <p>
element, you must create a text node first. This code creates a text node:
Then you must append the text node to the <p>
element:
Finally you must append the new element to an existing element.
This code finds an existing element:
This code appends the new element to the existing element:
Creating new HTML Elements - insertBefore()
The appendChild()
method in the previous example, appended the new element as the last child of the parent.
If you don't want that you can use the insertBefore()
method:
Example
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
<script>
const para = document.createElement("p");
const node = document.createTextNode("This is new.");
para.appendChild(node);
const element = document.getElementById("div1");
const child = document.getElementById("p1");
element.insertBefore(para, child);
</script>
Removing Existing HTML Elements
To remove an HTML element, use the remove()
method:
Example
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
<script>
const elmnt = document.getElementById("p1"); elmnt.remove();
</script>
Example Explained
The HTML document contains a <div>
element with two child nodes (two <p>
elements):
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
Find the element you want to remove:
Then execute the remove() method on that element:
The remove()
method does not work in older browsers, see the example below on how to use removeChild()
instead.
Removing a Child Node
For browsers that does not support the remove()
method, you have to find the parent node to remove an element:
Example
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
<script>
const parent = document.getElementById("div1");
const child = document.getElementById("p1");
parent.removeChild(child);
</script>
Example Explained
This HTML document contains a <div>
element with two child nodes (two <p>
elements):
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
Find the element with id="div1"
:
Find the <p>
element with id="p1"
:
Remove the child from the parent:
Here is a common workaround: Find the child you want to remove, and use its parentNode
property to find the parent:
child.parentNode.removeChild(child);
Replacing HTML Elements
To replace an element to the HTML DOM, use the replaceChild()
method:
Example
<p id="p1">This is a paragraph.</p>
<p id="p2">This is another paragraph.</p>
</div>
<script>
const para = document.createElement("p");
const node = document.createTextNode("This is new.");
para.appendChild(node);
const parent = document.getElementById("div1");
const child = document.getElementById("p1");
parent.replaceChild(para, child);
</script>
Practice Excercise Practice now
JavaScript HTML DOM Collections
The HTMLCollection Object
The getElementsByTagName()
method returns an HTMLCollection
object.
An HTMLCollection
object is an array-like list (collection) of HTML elements.
The following code selects all <p>
elements in a document:
Example
The elements in the collection can be accessed by an index number.
To access the second <p>
element you can write:
Note: The index starts at 0.
HTML HTMLCollection Length
The length
property defines the number of elements in an HTMLCollection
:
Example
The length
property is useful when you want to loop through the elements in a collection:
Example
Change the text color of all <p>
elements:
for (let i = 0; i < myCollection.length; i++) {
myCollection[i].style.color = "red";
}
An HTMLCollection is NOT an array!
An HTMLCollection may look like an array, but it is not.
You can loop through the list and refer to the elements with a number (just like an array).
However, you cannot use array methods like valueOf(), pop(), push(), or join() on an HTMLCollection.
Practice Excercise Practice now
JavaScript HTML DOM Node Lists
The HTML DOM NodeList Object
A NodeList
object is a list (collection) of nodes extracted from a document.
A NodeList
object is almost the same as an HTMLCollection
object.
Some (older) browsers return a NodeList object instead of an HTMLCollection for methods like getElementsByClassName()
.
All browsers return a NodeList object for the property childNodes
.
Most browsers return a NodeList object for the method querySelectorAll()
.
The following code selects all <p>
nodes in a document:
Example
Note: The index starts at 0.
HTML DOM Node List Length
The length
property defines the number of nodes in a node list:
Example
The length
property is useful when you want to loop through the nodes in a node list:
Example
for (let i = 0; i < myNodelist.length; i++) {
myNodelist[i].style.color = "red";
}
The Difference Between an HTMLCollection and a NodeList
An HTMLCollection
(previous chapter) is a collection of HTML elements.
A NodeList
is a collection of document nodes.
A NodeList and an HTML collection is very much the same thing.
Both an HTMLCollection object and a NodeList object is an array-like list (collection) of objects.
Both have a length property defining the number of items in the list (collection).
Both provide an index (0, 1, 2, 3, 4, ...) to access each item like an array.
HTMLCollection items can be accessed by their name, id, or index number.
NodeList items can only be accessed by their index number.
Only the NodeList object can contain attribute nodes and text nodes.
A node list is not an array!
A node list may look like an array, but it is not.
You can loop through the node list and refer to its nodes like an array.
However, you cannot use Array Methods, like valueOf(), push(), pop(), or join() on a node list.
Practice Excercise Practice now